资源简介
采用c++面向对象的思想设计的格斗类游戏,包括玩家类,继承自玩家类的弓箭手、法师和战士类,以及存放人物道具的背包类。
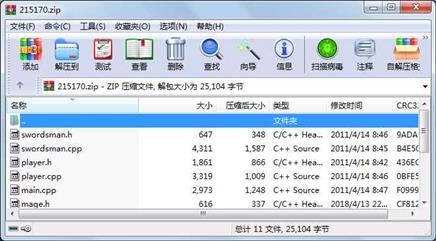
代码片段和文件信息
//=======================
// archer.cpp
//=======================
#include “archer.h“
Archer::Archer(int lv_in string name_in)
{
role = ar; // enumerate type of job
LV = lv_in;
name = name_in;
// Initialising the character‘s properties based on his level
HPmax = 150 + 6 * (LV - 1); // HP increases 6 point2 per level
HP = HPmax;
MPmax = 75 + 2 * (LV - 1); // MP increases 2 points per level
MP = MPmax;
AP = 25 + 8 * (LV - 1); // AP increases 8 points per level
DP = 25 + 2 * (LV - 1); // DP increases 2 points per level
speed = 25 + 2 * (LV - 1); // speed increases 2 points per level
playerdeath = 0;
EXP = LV*LV * 75;
bag.set(lv_in lv_in);
}
void Archer::isLevelUp()
{
if (EXP >= LV*LV * 75)
{
LV++;
AP += 8;
DP += 2;
HPmax += 6;
MPmax += 2;
speed += 2;
cout << name << “ Level UP!“ << endl;
cout << “HP improved 6 points to “ << HPmax << endl;
cout << “MP improved 2 points to “ << MPmax << endl;
cout << “Speed improved 2 points to “ << speed << endl;
cout << “AP improved 8 points to “ << AP << endl;
cout << “DP improved 2 points to “ << DP << endl;
system(“pause“);
isLevelUp(); // recursively call this function so the character can level up multiple times if got enough exp
}
}
bool Archer::attack(player &p)
{
double HPtemp = 0; // opponent‘s HP decrement
double EXPtemp = 0; // player obtained exp
double hit = 1.2; // attach factor probably give critical attack
srand((unsigned)time(NULL)); // generating random seed based on system time
// If speed greater than opponent you have some possibility to do double attack
if ((speed > p.speed) && (rand() % 100 < (speed - p.speed))) // rand()%100 means generates a number no greater than 100
{
HPtemp = (int)((1.0*AP / p.DP)*AP * 5 / (rand() % 4 + 10)); // opponent‘s HP decrement calculated based their AP/DP and uncertain chance
cout << name << “‘s quick strike hit “ << p.name << “ “ << p.name << “‘s HP decreased “ << HPtemp << endl;
p.HP = int(p.HP - HPtemp);
EXPtemp = (int)(HPtemp*1.2);
}
// If speed smaller than opponent the opponent has possibility to evade
if ((speed < p.speed) && (rand() % 50 < 1))
{
cout << name << “‘s attack has been evaded by “ << p.name << endl;
system(“pause“);
return 1;
}
// 10% chance give critical attack
if (rand() % 100 <= 10)
{
hit = 1.7;
cout << “Critical attack: “;
}
// Normal attack
HPtemp = (int)((1.0*AP / p.DP)*AP * 5 / (rand() % 4 + 10));
cout << name << “ uses bash “ << p.name << “‘s HP decreases “ << HPtemp << endl;
EXPtemp = (int)(EXPtemp + HPtemp*1.2);
p.HP = (int)(p.HP - HPtemp);
cout << name << “ obtained “ << EXPtemp << “ experience.“ << endl;
EXP = (int)(EXP + EXPtemp);
system(“pause“);
return 1; // Attack success
}
bool Archer::specialatt(player &p)
{
if (MP < 40)
{
cout << “You don‘t have enough magic points!“ << endl;
system(“pause“);
re
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 4586 2018-04-13 21:42 archer.cpp
文件 646 2018-04-13 22:07 archer.h
文件 844 2011-04-14 08:46 container.cpp
文件 682 2011-04-14 08:40 container.h
文件 4619 2018-04-13 21:29 mage.cpp
文件 616 2018-04-13 22:57 mage.h
文件 2973 2011-04-14 08:47 main.cpp
文件 3319 2011-04-14 08:46 pla
文件 1861 2011-04-14 08:42 pla
文件 4311 2011-04-14 08:45 swordsman.cpp
文件 647 2011-04-14 08:46 swordsman.h
- 上一篇:大数计算器
- 下一篇:c语言实现的自动贩卖机程序
相关资源
- 工程库实现面向对象编程
- C语言面向对象编程
- [BUPT]面向对象程序设计C++ - 平时作业
- 《面向对象的程序设计语言——C++》
- 谭浩强《C++面向对象程序设计》实验
- 面向对象编程MFC综合实验代码
- 基于qt的c++编写的贪吃蛇游戏
- c++面向对象图书管理系统
- 清大谭浩强教授的C++教程
- C++ 面向对象程序设计(第七版) 周靖
- 数据结构用面向对象的方法与C++语言
- C++教程 面向对象编程 清华大学出版
- C++面向对象程序设计 经典例题 附练习
- 黄维通Visual C++面向对象与可视化程序
- 黄维通Visual C++面向对象与可视化程序
- C++面向对象程序设计题解与上机指导
- VC++面向对象与可视化程序设计第三版
- Visual C++面向对象与可视化程序设计
- C++面向对象程序设计龚晓庆课后习题
- C++:面向对象程序设计陈良银 李涛
- 数据结构用面向对象方法与c++语言描
- 给力仿真电梯MFC源码含报告
- C++面向对象程序设计实验
- c++对战游戏
- C++面向对象程序设计教程-陈维兴-清华
- c++面向对象程序设计教程第三版陈维
- 倍福面向对象编程手册 TWincat3
- c++面向对象程序设计_杜茂康_课后答案
- 2D格斗游戏,C语言实现
- 五邑大学面向对象C++之MFC实验 学生成
评论
共有 条评论