资源简介
线程池 c++
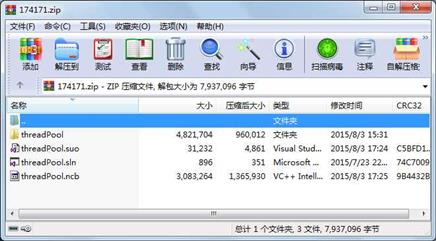
代码片段和文件信息
#include “stdafx.h“
#include “mem_pool.h“
//#include “../auto_lock.h“
//--------------------------------------------MemBlock----------------------------------------------------
#define LOG_TAG “mem pool“
FixedMemBlock::FixedMemBlock(UINT unitSize USHORT unitCount) :
m_blockTotalSize(unitSize * unitCount)
m_freeUnitCount(unitCount - 1)
m_firstFreeIndex(1)
m_pNextBlock(NULL)
m_unitSize(unitSize)
{
char *p = m_firstByte;
//the min index is 1 max index is (unitCount - 1)
//0th unit points to 1th unit 1th points to 2th...(unitCount-2)th points to (unitCount-1)
for (int i = 1; i <= unitCount - 1; ++i)
{
(*(USHORT *)p) = i;
p += unitSize;
}
}
FixedMemBlock::~FixedMemBlock()
{}
void *FixedMemBlock::getFirstFreeUnit()
{
void *pRet = NULL;
if(m_freeUnitCount > 0)
{
pRet = m_firstByte + ((UINT)m_firstFreeIndex) * m_unitSize;
#ifdef FIXEDMEMPOOL_DEBUG
PRINT_I(LOG_TAG “alloc unit %i %i from %i“ m_firstFreeIndex pRet this);
#endif
m_firstFreeIndex = *(USHORT *)pRet;
m_freeUnitCount--;
#ifdef FIXEDMEMPOOL_DEBUG
PRINT_I(LOG_TAG “next unit %i“ m_firstFreeIndex);
PRINT_I(LOG_TAG “current unit count %i“ m_freeUnitCount);
PRINT_I(LOG_TAG “--------------------------“);
#endif
}
return pRet;
}
bool FixedMemBlock::containsUnit(void *addr)
{
ULONG l = (ULONG)addr;
return (l >= (ULONG)(m_firstByte) && l < (ULONG)(m_firstByte + m_blockTotalSize));
}
void FixedMemBlock::releaseUnit(void *p)
{
//when invoke this method should guarantee that p definitely belongs to this block
//so it‘s private not allow user to invoke it
//write the pre-free index to the head of the unit
*(USHORT *)p = m_firstFreeIndex;
m_firstFreeIndex = (USHORT)(((ULONG)p - (ULONG)(m_firstByte)) / m_unitSize);
m_freeUnitCount++;
#ifdef FIXEDMEMPOOL_DEBUG
PRINT_I(LOG_TAG “release unit %i from %i“ m_firstFreeIndex this);
PRINT_I(LOG_TAG “current unit count %i“ m_freeUnitCount);
#endif
}
void * FixedMemBlock::operator new(size_t UINT unitSize UINT unitCount)
{
void *ret = ::operator new(sizeof(FixedMemBlock) + unitSize * unitCount);
#ifdef FIXEDMEMPOOL_DEBUG
PRINT_I(LOG_TAG “alloc new block %i“ ret);
#endif
return ret;
}
void FixedMemBlock::operator delete(void *p UINT unitSize UINT unitCount)
{
if (p)::operator delete(p);
}
void FixedMemBlock::operator delete(void *p size_t)
{
#ifdef FIXEDMEMPOOL_DEBUG
PRINT_I(LOG_TAG “release block %i“ p);
#endif
if (p)::operator delete(p);
}
//--------------------------------------------MemPool----------------------------------------------------
FixedMemPool::FixedMemPool():
m_pBlockHead(NULL)
m_blockCount(0)
m_autoReleaseFreeBlock(false)
m_maxReserveBlocksCount(0)
m_totalAllocSize(0)
m_isInited(false)
m_unitSize(0)
m_unitCountPerBlock(0)
{
}
FixedMemPool::FixedMemPool(UINT unitSize USHORT unitCountPerBlock) :
m_pBlockHead(NULL)
m_blockCount(0)
m_autoReleaseFreeBl
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2015-08-03 15:31 threadPool\
文件 3083264 2015-08-03 17:25 threadPool.ncb
文件 896 2015-07-23 22:02 threadPool.sln
文件 31232 2015-08-03 17:24 threadPool.suo
目录 0 2015-08-03 15:31 threadPool\Debug\
文件 6706 2015-08-03 15:31 threadPool\Debug\BuildLog.htm
文件 20691 2015-07-24 00:57 threadPool\Debug\mem_pool.obj
文件 65 2015-08-03 15:31 threadPool\Debug\mt.dep
文件 11917 2015-07-23 22:12 threadPool\Debug\stdafx.obj
文件 68561 2015-07-28 22:17 threadPool\Debug\thread.obj
文件 663 2015-07-23 22:12 threadPool\Debug\threadPool.exe.em
文件 728 2015-07-23 22:12 threadPool\Debug\threadPool.exe.em
文件 621 2015-08-03 15:31 threadPool\Debug\threadPool.exe.intermediate.manifest
文件 60963 2015-08-03 15:31 threadPool\Debug\threadPool.obj
文件 3211264 2015-07-23 22:12 threadPool\Debug\threadPool.pch
文件 353307 2015-08-03 15:29 threadPool\Debug\ThreadPoolExecutor.obj
文件 666624 2015-08-03 15:31 threadPool\Debug\vc90.idb
文件 389120 2015-08-03 15:31 threadPool\Debug\vc90.pdb
文件 6986 2015-07-24 00:57 threadPool\mem_pool.cpp
文件 2413 2015-07-24 00:57 threadPool\mem_pool.h
文件 1201 2015-07-23 22:02 threadPool\ReadMe.txt
文件 215 2015-07-23 22:02 threadPool\stdafx.cpp
文件 233 2015-07-23 22:02 threadPool\stdafx.h
文件 498 2015-07-23 22:02 threadPool\targetver.h
文件 2441 2015-07-24 00:58 threadPool\thread.cpp
文件 1624 2015-07-23 22:04 threadPool\thread.h
文件 785 2015-08-03 15:31 threadPool\threadPool.cpp
文件 4901 2015-07-24 00:55 threadPool\threadPool.vcproj
文件 1413 2015-08-03 17:24 threadPool\threadPool.vcproj.zhang-PC.zhang.user
文件 5553 2015-08-03 15:28 threadPool\ThreadPoolExecutor.cpp
文件 2211 2015-07-24 00:52 threadPool\ThreadPoolExecutor.h
............此处省略0个文件信息
相关资源
- 国际象棋的qt源代码
- C++中头文件与源文件的作用详解
- C++多线程网络编程Socket
- VC++ 多线程文件读写操作
- 利用C++哈希表的方法实现电话号码查
- 移木块游戏,可以自编自玩,vc6.0编写
- C++纯文字DOS超小RPG游戏
- VC++MFC小游戏实例教程(实例)+MFC类库
- 连铸温度场计算程序(C++)
- 6自由度机器人运动学正反解C++程序
- Em算法(使用C++编写)
- libstdc++-4.4.7-4.el6.i686.rpm
- VC++实现CMD命令执行与获得返回信息
- 白话C++(全)
- C++标准库第1、2
- 大数类c++大数类
- C++语言编写串口调试助手
- c++素数筛选法
- C++ mqtt 用法
- 商品库存管理系统 C++ MFC
- c++ 多功能计算器
- C++17 In Detail
- 嵌入式QtC++编程课件
- 颜色识别形状识别STM103嵌入式代码
- c++ 邮件多附件群发
- c++ 透明代理(hookproxy)
- mfc 调用redis
- FTP客户端源码(c++)
- c++ 画图(14Qt-XPS)
- c++多边形交并差运算
评论
共有 条评论