资源简介
根据每一行的字符串长度进行排序,长度相同的根据字符串优先级进行排序
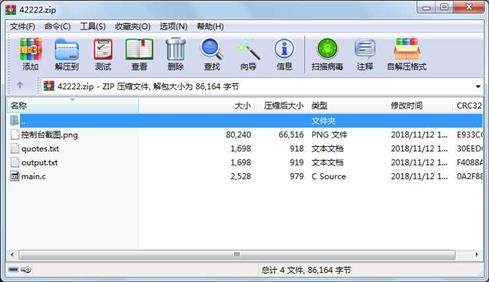
代码片段和文件信息
#include
#include
#include
const unsigned int MAX_SIZE = 100;
typedef unsigned int uint;
// This function will be used to swap “pointers“.
void swap(char** char**);
// Bubble sort function here.
void bubbleSort(char** uint);
// Read quotes from quotes.txt file file and add them to array. Adjust the size as well!
// Note: size should reflect the number of quotes in the array/quotes.txt file!
void read_in(char** uint*);
// Print the quotes using array of pointers.
void print_out(char** uint);
// Save the sorted quotes in the output.txt file
void write_out(char** uint);
// Free memory!
void free_memory(char** uint);
int main() {
// Create array of pointers. Each pointer should point to heap memory where
// each quote is kept. I.e. arr[0] points to quote N1 saved on the heap.
char *arr[MAX_SIZE];
// Number of quotes in the file quotes.txt. Must be adjusted when the file is read!
uint size = MAX_SIZE;
read_in(arr &size);
printf(“--- Input:\n“);
print_out(arr size);
bubbleSort(arr size);
printf(“--- Output:\n“);
print_out(arr size);
write_out(arr size);
free_memory(arr size);
return (0);
}
void swap(char** a char** b)
{
char* tmp = *a;
*a = *b;
*b = tmp;
}
void bubbleSort(char** arr uint size)
{
uint i j;
for (i = 0; i < size - 1; i++)
for (j = 0; j < size - i - 1; j++)
if (strlen(arr[j]) > strlen(arr[j + 1]) ||
strlen(arr[j]) == strlen(arr[j + 1]) && strcmp(arr[j]arr[j + 1])>0)
swap(&arr[j] &arr[j + 1]);
}
void read_in(char** arr uint* size)
{
FILE *fin = fopen(“quotes.txt“ “r“);
if (!fin) {
printf(“can‘t open file!\n“);
return;
}
*size = 0;
char line[200];
while (fgets(line200fin) != 0)
{
if (*size == MAX_SIZE) {
printf(“file is too long!\n“);
return;
}
int len = strlen(line);
char* quote = (char*)malloc(sizeof(char)*len);
if (!quote) {
printf(“malloc error!\n“);
return;
}
memccpy(quote line sizeof(char) len);
quote[len - 1] = ‘\0‘;
arr[(*size)++] = quote;
}
fclose(fin);
}
void print_out(char** arr uint size)
{
for (uint i = 0; i < size; ++i)
printf(“%s\n“ arr[i]);
}
void write_out(char** arr uint size)
{
FILE *fout = fopen(“output.txt“ “w“);
for (uint i = 0; i < size; ++i)
fprintf(fout “%s\n“ arr[i]);
fclose(fout);
}
void free_memory(char** arr uint size)
{
for (uint i = 0; i < size; ++i)
free(arr[i]);
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 2528 2018-11-12 10:23 main.c
文件 1698 2018-11-12 10:21 output.txt
文件 1698 2018-11-12 10:12 quotes.txt
文件 80240 2018-11-12 10:21 控制台截图.png
- 上一篇:最低公共祖先算法实现
- 下一篇:判断两个数组的关系
评论
共有 条评论