资源简介
用C#实现的FFT和IFFT类,方便初学者进行学习,也可直接在代码里使用
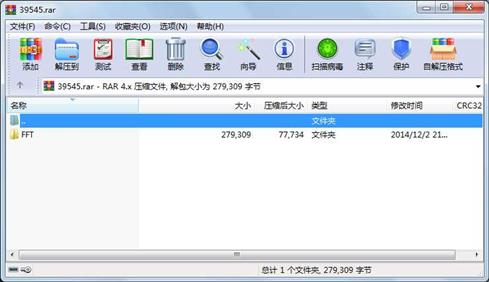
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace FFT
{
///
/// 复数类
///
class Complex
{
///
/// 默认构造函数
///
public Complex()
: this(0 0)
{
}
///
/// 只有实部的构造函数
///
/// 实部
public Complex(double real)
: this(real 0) { }
///
/// 由实部和虚部构造
///
/// 实部
/// 虚部
public Complex(double real double image)
{
this.real = real;
this.image = image;
}
private double real;
///
/// 复数的实部
///
public double Real
{
get { return real; }
set { real = value; }
}
private double image;
///
/// 复数的虚部
///
public double Image
{
get { return image; }
set { image = value; }
}
///重载加法
public static Complex operator +(Complex c1 Complex c2)
{
return new Complex(c1.real + c2.real c1.image + c2.image);
}
///重载减法
public static Complex operator -(Complex c1 Complex c2)
{
return new Complex(c1.real - c2.real c1.image - c2.image);
}
///重载乘法
public static Complex operator *(Complex c1 Complex c2)
{
return new Complex(c1.real * c2.real - c1.image * c2.image c1.image * c2.real + c1.real * c2.image);
}
///
/// 求复数的模
///
/// 模
public double ToModul()
{
return Math.Sqrt(real * real + image * image);
}
///
/// 重载ToString方法
///
/// 打印字符串
public override string ToString()
{
if (Real == 0 && Image == 0)
{
return string.Format(“{0}“ 0);
}
if (Real == 0 && (Image != 1 && Image != -1))
{
return string.Format(“{0} i“ Image);
}
if (Image == 0)
{
return string.Format(“{0}“ Real);
}
if (Image == 1)
{
return string.Format(“i“);
}
if (Image == -1)
{
return string.Format(“- i“);
}
if (Image < 0)
{
return string.Format(“{0} - {1} i“ Real -Image);
}
return string.Format(“{0} + {1} i
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 187 2014-12-02 20:29 FFT\FFT\App.config
文件 8192 2014-12-02 21:54 FFT\FFT\bin\Debug\FFT.exe
文件 187 2014-12-02 20:29 FFT\FFT\bin\Debug\FFT.exe.config
文件 24064 2014-12-02 21:54 FFT\FFT\bin\Debug\FFT.pdb
文件 22984 2014-12-02 21:58 FFT\FFT\bin\Debug\FFT.vshost.exe
文件 187 2014-12-02 20:29 FFT\FFT\bin\Debug\FFT.vshost.exe.config
文件 3180 2014-12-02 20:36 FFT\FFT\Complex.cs
文件 2624 2014-12-02 21:15 FFT\FFT\FFT.csproj
文件 1799 2014-12-02 21:44 FFT\FFT\FFTMain.cs
文件 4502 2014-12-02 21:38 FFT\FFT\FFT_IFFT.cs
文件 6442 2014-12-02 21:15 FFT\FFT\obj\Debug\DesignTimeResolveAssemblyReferencesInput.cache
文件 217 2014-12-02 21:58 FFT\FFT\obj\Debug\FFT.csproj.FileListAbsolute.txt
文件 8192 2014-12-02 21:54 FFT\FFT\obj\Debug\FFT.exe
文件 24064 2014-12-02 21:54 FFT\FFT\obj\Debug\FFT.pdb
文件 0 2014-12-02 20:29 FFT\FFT\obj\Debug\TemporaryGeneratedFile_036C0B5B-1481-4323-8D20-8F5ADCB23D92.cs
文件 0 2014-12-02 20:29 FFT\FFT\obj\Debug\TemporaryGeneratedFile_5937a670-0e60-4077-877b-f7221da3dda1.cs
文件 0 2014-12-02 20:29 FFT\FFT\obj\Debug\TemporaryGeneratedFile_E7A71F73-0F8D-4B9B-B56E-8E70B10BC5D3.cs
文件 1326 2014-12-02 20:29 FFT\FFT\Properties\AssemblyInfo.cs
文件 1377 2014-12-02 21:46 FFT\FFT.sln
..A..H. 39936 2014-12-02 22:52 FFT\FFT.v11.suo
文件 187 2014-12-02 21:45 FFT\FFT_Form\App.config
文件 523 2014-12-02 21:46 FFT\FFT_Form\AppMain.cs
文件 9728 2014-12-02 22:37 FFT\FFT_Form\bin\Debug\FFT_Form.exe
文件 187 2014-12-02 21:45 FFT\FFT_Form\bin\Debug\FFT_Form.exe.config
文件 24064 2014-12-02 22:37 FFT\FFT_Form\bin\Debug\FFT_Form.pdb
文件 22984 2014-12-02 22:37 FFT\FFT_Form\bin\Debug\FFT_Form.vshost.exe
文件 187 2014-12-02 21:45 FFT\FFT_Form\bin\Debug\FFT_Form.vshost.exe.config
文件 3810 2014-12-02 21:54 FFT\FFT_Form\FFT_Form.csproj
文件 2736 2014-12-02 22:52 FFT\FFT_Form\MainForm.cs
文件 5163 2014-12-02 22:02 FFT\FFT_Form\MainForm.Designer.cs
............此处省略37个文件信息
- 上一篇:C#服装仓库管理系统
- 下一篇:新生报到管理系统
相关资源
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
- C# 使用ListView控件实现图片浏览器(源
- C#简单窗体聊天程序
- C#指纹识别系统程序 报告
- c# 高校档案信息管理系统
- c#向word文件插入图片
- C#左侧导航菜单(动态生成)
评论
共有 条评论