-
大小: 61KB文件类型: .zip金币: 2下载: 0 次发布日期: 2022-06-24
- 语言: C#
- 标签: c# serialport sample
资源简介
http://blog.csdn.net/wuyazhe/archive/2010/05/27/5627253.aspx 博客文章的配套代码。希望能帮助到你。
vs2010工程,编码都只是用到vs2005的语法,可以反向升级。
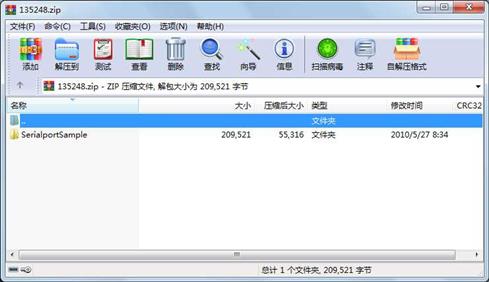
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO.Ports;
using System.Text.Regularexpressions;
namespace SerialportSample
{
public partial class SerialportSampleForm : Form
{
private SerialPort comm = new SerialPort();
private StringBuilder builder = new StringBuilder();//避免在事件处理方法中反复的创建,定义到外面。
private long received_count = 0;//接收计数
private long send_count = 0;//发送计数
private bool Listening = false;//是否没有执行完invoke相关操作
private bool Closing = false;//是否正在关闭串口,执行Application.DoEvents,并阻止再次invoke
private List buffer = new List(4096);//默认分配1页内存,并始终限制不允许超过
private byte[] binary_data_1 = new byte[9];//AA 44 05 01 02 03 04 05 EA
public SerialportSampleForm()
{
InitializeComponent();
}
//窗体初始化
private void Form1_Load(object sender EventArgs e)
{
//初始化下拉串口名称列表框
string[] ports = SerialPort.GetPortNames();
Array.Sort(ports);
comboPortName.Items.AddRange(ports);
comboPortName.SelectedIndex = comboPortName.Items.Count > 0 ? 0 : -1;
comboBaudrate.SelectedIndex = comboBaudrate.Items.IndexOf(“19200“);
//初始化SerialPort对象
comm.NewLine = “\r\n“;
comm.RtsEnable = true;//根据实际情况吧。
//添加事件注册
comm.DataReceived += comm_DataReceived;
}
void comm_DataReceived(object sender SerialDataReceivedEventArgs e)
{
if (Closing) return;//如果正在关闭,忽略操作,直接返回,尽快的完成串口监听线程的一次循环
try
{
Listening = true;//设置标记,说明我已经开始处理数据,一会儿要使用系统UI的。
int n = comm.BytesToRead;//先记录下来,避免某种原因,人为的原因,操作几次之间时间长,缓存不一致
byte[] buf = new byte[n];//声明一个临时数组存储当前来的串口数据
received_count += n;//增加接收计数
comm.Read(buf 0 n);//读取缓冲数据
/////////////////////////////////////////////////////////////////////////////////////////////////////////////
//<协议解析>
bool data_1_catched = false;//缓存记录数据是否捕获到
//1.缓存数据
buffer.AddRange(buf);
//2.完整性判断
while (buffer.Count >= 4)//至少要包含头(2字节)+长度(1字节)+校验(1字节)
{
//请不要担心使用>=,因为>=已经和><=一样,是独立操作符,并不是解析成>和=2个符号
//2.1 查找数据头
if (buffer[0] == 0xAA && buffer[1] == 0x44)
{
//2.2 探测缓存数据是否有一条数据的字节,如果不够,就不用费劲的做其他验证了
//前面已经限定了剩余长度>=4,那我们这里一定能访问到buffer[2]这个长度
int len = buffer[2];//数据长度
//数据完整判断第一步,长度是否足够
//len是数据段长度4个字节是while行注释的3部分长度
i
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2010-05-27 08:34 SerialportSample\
目录 0 2010-05-27 08:34 SerialportSample\SerialportSample\
文件 890 2010-05-16 02:19 SerialportSample\SerialportSample.sln
文件 19968 2010-05-27 11:27 SerialportSample\SerialportSample.suo
目录 0 2010-05-27 08:34 SerialportSample\SerialportSample\bin\
目录 0 2010-05-27 08:34 SerialportSample\SerialportSample\bin\Debug\
文件 17408 2010-05-27 09:25 SerialportSample\SerialportSample\bin\Debug\SerialportSample.exe
文件 38400 2010-05-27 09:25 SerialportSample\SerialportSample\bin\Debug\SerialportSample.pdb
文件 11600 2010-05-27 10:21 SerialportSample\SerialportSample\bin\Debug\SerialportSample.vshost.exe
文件 490 2010-03-17 22:39 SerialportSample\SerialportSample\bin\Debug\SerialportSample.vshost.exe.manifest
文件 11471 2010-05-27 10:20 SerialportSample\SerialportSample\Form1.cs
文件 16740 2010-05-27 08:52 SerialportSample\SerialportSample\Form1.Designer.cs
文件 5817 2010-05-27 08:52 SerialportSample\SerialportSample\Form1.resx
目录 0 2010-05-27 08:34 SerialportSample\SerialportSample\obj\
目录 0 2010-05-27 08:34 SerialportSample\SerialportSample\obj\x86\
目录 0 2010-05-27 10:21 SerialportSample\SerialportSample\obj\x86\Debug\
文件 4440 2010-05-27 10:21 SerialportSample\SerialportSample\obj\x86\Debug\DesignTimeResolveAssemblyReferences.cache
文件 6274 2010-05-27 09:25 SerialportSample\SerialportSample\obj\x86\Debug\DesignTimeResolveAssemblyReferencesInput.cache
文件 642 2010-05-27 09:08 SerialportSample\SerialportSample\obj\x86\Debug\GenerateResource.read.1.tlog
文件 1614 2010-05-27 09:08 SerialportSample\SerialportSample\obj\x86\Debug\GenerateResource.write.1.tlog
文件 2099 2010-05-27 10:21 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.csproj.FileListAbsolute.txt
文件 17408 2010-05-27 09:25 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.exe
文件 38400 2010-05-27 09:25 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.pdb
文件 180 2010-05-27 09:08 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.Properties.Resources.resources
文件 180 2010-05-27 09:08 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.SerialportSampleForm.resources
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\obj\x86\Debug\TempPE\
文件 523 2010-05-16 03:24 SerialportSample\SerialportSample\Program.cs
目录 0 2010-05-27 08:34 SerialportSample\SerialportSample\Properties\
文件 1468 2010-05-16 02:19 SerialportSample\SerialportSample\Properties\AssemblyInfo.cs
文件 2858 2010-05-16 02:19 SerialportSample\SerialportSample\Properties\Resources.Designer.cs
文件 5612 2010-05-16 02:19 SerialportSample\SerialportSample\Properties\Resources.resx
............此处省略3个文件信息
- 上一篇:C#图片/相册管理程序代码
- 下一篇:c#网上选课系统
相关资源
- C#解析HL7消息的库135797
- C# OCR数字识别实例,采用TessnetOcr,对
- 考试管理系统 - C#源码
- asp.net C#购物车源代码
- C#实时网络流量监听源码
- C#百度地图源码
- Visual C#.2010从入门到精通配套源程序
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
评论
共有 条评论