资源简介
本项目为我的C#作业,完整的中国象棋游戏,单机版,优质的画面,清晰完整代码注释,真实完整,非常有趣,下载了绝不后悔!!!
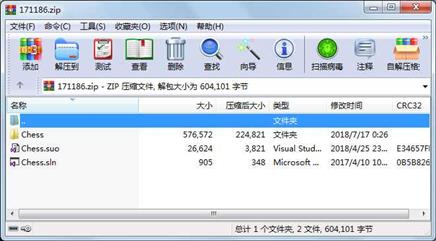
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
//添加命名空间
using System.IO;
using System.Media;
using System.Diagnostics;
namespace Chess
{
//棋子枚举类型
public enum Piece
{
无子 = 0
红车 = 1 红马 = 2 红相 = 3 红士 = 4 红帅 = 5 红炮 = 6 红卒 = 7
蓝车 = 8 蓝马 = 9 蓝象 = 10 蓝士 = 11 蓝将 = 12 蓝炮 = 13 蓝兵 = 14
}
//走棋方枚举类型
public enum Player
{
无 = 0 红 = 1 蓝 = 2
}
//主窗口类
public partial class FormMain : Form
{
//保存象棋棋盘的所有棋子值(棋盘共10行9列)
private Piece[] _chess = new Piece[10 9];
//保存所有的走棋步骤(List对象)
private List _stepList = new List();
//保存棋盘(细框)的左上角坐标
private Point _leftTop = new Point(60 60);
//保存棋盘格子的行高和列宽
private int _rowHeight = 60;
private int _colWidth = 60;
//保存棋子半径
private int _pieceR = 29;
//保存拾起的棋子值(默认为“无子“,即目前没有拾起棋子)
private Piece _pickChess = Piece.无子;
//保存拾起棋子的行号和列号
private int _pickRow = 0;
private int _pickCol = 0;
//保存落下棋子的行号和列号
private int _dropRow = 0;
private int _dropCol = 0;
//保存当前鼠标所在点坐标
private Point _curMousePoint = new Point(0 0);
//保存棋盘桌面位图
private Bitmap _deskBmp = new Bitmap(“desktop.jpg“);
//保存红蓝双方14种棋子位图(为了与Piece枚举类型值对应,_pieceBmp[0]舍弃不用)
private Bitmap[] _pieceBmp = new Bitmap[15];
//保存红方头像位图
private Bitmap _redBmp = new Bitmap(“红方头像.bmp“);
//保存蓝方头像位图
private Bitmap _blueBmp = new Bitmap(“蓝方头像.bmp“);
//保存当前的走棋方
private Player _curPlayer = Player.无;
//保存项目“.exe“文件的所在路径
private string _exePath;
//自定义类方法:绘制棋盘
public void DrawBoard(Graphics g)
{
//绘制棋盘桌面
g.DrawImage(_deskBmp new Point(0 0));
//创建粗画笔和细画笔
Pen thickPen = new Pen(Color.Black 6);
Pen thinPen = new Pen(Color.Black 2);
//绘制棋盘外围的粗框
int gap = (int)(_rowHeight*0.15);
g.DrawRectangle(thickPen new Rectangle(_leftTop.X - gap _leftTop.Y - gap _colWidth * 8 + 2 * gap _rowHeight * 9 + 2 * gap));
//绘制棋盘的10条横线(10行)
for (int row = 1; row <= 10; row++)
{
g.DrawLine(thinPen new Point(_leftTop.X _leftTop.Y + _rowHeight * (row - 1))
new Point(_leftTop.X + 8 * _colWidth _leftTop.Y + _rowHeight * (row - 1)));
}
//绘制棋盘的9条竖线(9列)
for (int col = 1; col <= 9; col++)
{
//绘制棋盘上半部分的竖线
g.DrawLine(thinPen new Point(_leftTop.X + (col - 1) * _colWidth _leftTop.Y)
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 905 2017-04-10 10:29 Chess.sln
文件 26624 2018-04-25 23:01 Chess.suo
目录 0 2018-07-17 00:26 Chess\
目录 0 2018-07-17 00:26 Chess\bin\
目录 0 2018-07-17 00:26 Chess\bin\Debug\
文件 29184 2017-04-24 13:43 Chess\bin\Debug\Chess.exe
文件 73216 2017-04-24 13:43 Chess\bin\Debug\Chess.pdb
文件 14328 2018-04-25 22:54 Chess\bin\Debug\Chess.vshost.exe
文件 490 2009-06-11 05:14 Chess\bin\Debug\Chess.vshost.exe.manifest
文件 63289 2011-02-22 21:37 Chess\bin\Debug\desktop.jpg
目录 0 2018-07-17 00:26 Chess\bin\Debug\Sounds\
文件 127076 2010-07-30 11:01 Chess\bin\Debug\Sounds\begin.wav
文件 3362 2010-07-30 11:01 Chess\bin\Debug\Sounds\drop.wav
文件 16992 2010-07-30 11:01 Chess\bin\Debug\Sounds\eat.wav
文件 43402 2010-07-30 11:01 Chess\bin\Debug\Sounds\over.wav
文件 4560 2007-12-28 09:52 Chess\bin\Debug\红兵.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\红士.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\红帅.bmp
文件 5046 1999-11-04 14:46 Chess\bin\Debug\红方头像.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\红炮.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\红相.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\红车.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\红马.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\蓝兵.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\蓝士.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\蓝帅.bmp
文件 5046 1999-11-04 14:43 Chess\bin\Debug\蓝方头像.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\蓝炮.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\蓝象.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\蓝车.bmp
文件 4560 2007-12-28 09:52 Chess\bin\Debug\蓝马.bmp
............此处省略23个文件信息
相关资源
- EQ2008LEDc#开发实例
- 北大青鸟ACCP8.0S1使用C#开发数据库应用
- C#开发的OCR识别程序源码
- C#开发实例大全(提高卷)源码
- 基于C#开发OPC客户端
- C#开发的一个数据显示界面程序(Li
- C#开发的看盘小工具
- C#开发调用百度地图
- asp.netc#开发规范
- 酒店电子管理系统winform源代码(附数
- c#开发的WebFormDesigner
- C#开发 CAD 编程基础
- 用C#开发与Domino交互的应用原创
- 基于C#开发的企业工资管理系统
- c#影院系统大作业,班评第一名
- C#企业电话客服系统215910
- 科大讯飞 语音唤醒及语音听写服务
- 基于RFID的学生门禁系统C#、mysql、RF
- C#开发的聊天小程序
- 基于C#开发的音乐播放器
- 基于C#开发的迷你GIS系统
- C#开发影院售票系统
- C#开发的医院挂号管理系统
- C#开发的一款百宝箱
- C#开发无人机地面站
- ArcGIS_Engine_C#开发教程+源码超值
- c#开发的药品销售系统
- 基于C#开发的EMS
- C#开发的小区物业管理系统
- 电子病历源码纯c#开发
评论
共有 条评论