资源简介
课程资源分享,C#作业——大学生社团管理系统。根据.net三层架构设计。开发平台:vs2010,SQL serve 2008
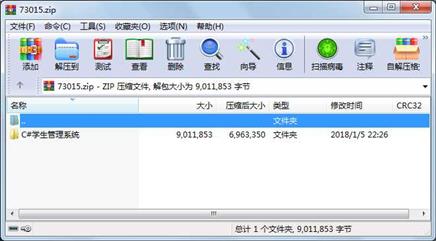
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using DAL;
using Model;
namespace BLL
{
///
/// 功能:提供对Club的业务逻辑管理
/// 开发者:曹萍萍
/// 更新日期:2017-11-08
///
public class ClubBLL
{
ClubDAL clubdal = new ClubDAL();
#region 查询操作
///
/// 功能:查询社团信息
///
/// 返回查询结果表
public DataTable GetClubList()
{
return clubdal.GetClubList();
}
///
/// 功能:根据社团名称模糊查询
///
/// 根据社团名称查询
/// 返回查询结果表
public DataTable GetClubListByName(string name)
{
return clubdal.GetClubListByName(name);
}
///
/// 功能:显示选中单元格的社团详情
///
/// 根据社团名称查询
/// 返回查询结果表
public Club GetClubByName(string name)
{
return clubdal.GetClubByName(name);
}
#endregion
#region 非查询操作
///
/// 功能:实现对Club的添加
///
/// 社团对象
/// 返回成功或失败
public int AddClub(Club c) //添加社团
{
Club club = clubdal.GetClubByName(c.ClubName);
//Student student = memberdal.GetMemberListByID(s.StudentID);
if (club == null)
{
if (clubdal.AddClub(c))
{
return 1; //添加成功
}
else
{
return 0; //添加失败
}
}
else
{
return -1; //名字重复
}
}
///
/// 功能:修改社团信息
///
/// 待修改社团名称
/// 返回成功或失败
public bool UpdateClub(Club c) //修改社团
{
return clubdal.UpdateClub(c);
}
///
/// 功能:删除社团信息
///
/// 待删除社团名称
/// 返回成功或失败
public bool DeleteClub(string clubname) //删除社团
{
return clubdal.DeleteClub(clubname);
}
#endregion
}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2018-01-05 22:26 C#学生管理系统\
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\BLL\
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\BLL\bin\
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\BLL\bin\Debug\
文件 6656 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\bin\Debug\BLL.dll
文件 28160 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\bin\Debug\BLL.pdb
文件 15360 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\bin\Debug\DAL.dll
文件 34304 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\bin\Debug\DAL.pdb
文件 8704 2017-11-13 03:36 C#学生管理系统\StudentClubMis02\BLL\bin\Debug\Model.dll
文件 32256 2017-11-13 03:36 C#学生管理系统\StudentClubMis02\BLL\bin\Debug\Model.pdb
目录 0 2017-10-25 10:36 C#学生管理系统\StudentClubMis02\BLL\bin\Release\
文件 2913 2017-11-13 01:18 C#学生管理系统\StudentClubMis02\BLL\BLL.csproj
文件 2923 2017-11-13 01:56 C#学生管理系统\StudentClubMis02\BLL\ClubBLL.cs
文件 1103 2017-11-13 01:57 C#学生管理系统\StudentClubMis02\BLL\ClubMemberBLL.cs
文件 706 2017-11-13 01:58 C#学生管理系统\StudentClubMis02\BLL\DepartmentBLL.cs
文件 666 2017-11-13 01:58 C#学生管理系统\StudentClubMis02\BLL\GradeBLL.cs
文件 2947 2017-11-08 08:51 C#学生管理系统\StudentClubMis02\BLL\MemberBLL.cs
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\BLL\obj\
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\BLL\obj\Debug\
文件 523 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\obj\Debug\BLL.csproj.FileListAbsolute.txt
文件 7745 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\obj\Debug\BLL.csprojResolveAssemblyReference.cache
文件 6656 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\obj\Debug\BLL.dll
文件 28160 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\obj\Debug\BLL.pdb
文件 5794 2017-11-13 03:37 C#学生管理系统\StudentClubMis02\BLL\obj\Debug\DesignTimeResolveAssemblyReferencesInput.cache
目录 0 2017-10-25 10:35 C#学生管理系统\StudentClubMis02\BLL\obj\Debug\TempPE\
文件 720 2017-11-13 01:59 C#学生管理系统\StudentClubMis02\BLL\ProfessionBLL.cs
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\BLL\Properties\
文件 1338 2017-10-25 10:35 C#学生管理系统\StudentClubMis02\BLL\Properties\AssemblyInfo.cs
文件 2097 2017-11-13 01:59 C#学生管理系统\StudentClubMis02\BLL\UserBLL.cs
目录 0 2017-11-13 03:38 C#学生管理系统\StudentClubMis02\DAL\
............此处省略229个文件信息
- 上一篇:深度学习 C#
- 下一篇:c#winform实现局域网内文件的拷贝
相关资源
- ASP.NET实验室预约管理系统
- C#百度地图源码
- Visual C#.2010从入门到精通配套源程序
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 020ASP.NET车辆综合管理系统.zip
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
- C# 使用ListView控件实现图片浏览器(源
- C#简单窗体聊天程序
评论
共有 条评论