资源简介
C# 窗体 仿做俄罗斯方块 游戏 源码例子
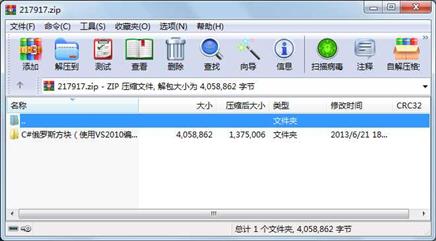
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Drawing;
namespace HuaDaBlock
{
class Block
{
#region 字段与属性
//单元格像素
private int squarePixel;
public int SquarePixel
{
get { return squarePixel; }
set { squarePixel = value; }
}
//单元格颜色
private Color squareColor;
public Color SquareColor
{
get { return squareColor; }
set { squareColor = value; }
}
//背景色
private Color backColor;
public Color BackColor
{
get { return backColor; }
set { backColor = value; }
}
//砖块中心格在游戏窗口中的绝对坐标(中心格在砖块框架中的相对坐标为(00))
public int XPost
{
get;
set;
}
public int YPost
{
get;
set;
}
//所有组成砖块的单元格在框架中的相对坐标该集合决定了砖块的样式
public List SquarePointList
{
get;
set;
}
////砖块种类的集合
//public BlockTypeInfo Types
//{
// get;
// set;
//}
#endregion
#region 构造函数
public Block()
{
}
public Block(int pixelColor sColor Color bColor List pList)
{
this.squarePixel = pixel;
this.squareColor = sColor;
this.backColor = bColor;
this.SquarePointList = pList;
}
#endregion
//获取框架中的单元格相对于游戏窗口中的单元格
protected Rectangle GetWindowSquare(Point p)
{
//位于游戏窗口中的x坐标
int x = (XPost + p.X) * (squarePixel + 2);
//位于游戏窗口中的y坐标
int y = (YPost + p.Y) * (squarePixel + 2);
return new Rectangle(x y squarePixel squarePixel);
}
//根据SquarePointList中的单元格信息在游戏窗口中绘制砖块
public void DrawBlock(Graphics board)
{
SolidBrush brush = new SolidBrush(squareColor);
foreach (Point item in SquarePointList)
{
////线程同步锁lock,使用的定时器多线程,需要上一把锁
lock (board)
{
board.FillRectangle(brush GetWindowSquare(item));
}
}
}
//清除原有方块
public void EraseOld(Graphics board)
{
SolidBrush brush = new SolidBrush(backColor);
foreach (Point item in SquarePointList)
{
////线程同步锁lock,使用的定时器多线程,需要上一把锁
lock (board)
{
board.FillRectangle(brush GetWindowSquare(item));
}
}
}
}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2013-06-21 18:54 C#俄罗斯方块(使用VS2010编写)\
目录 0 2013-06-21 18:54 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\
目录 0 2013-06-21 18:54 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\
文件 3271 2013-03-12 00:35 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Block.cs
文件 3360 2013-03-11 22:04 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Brickyard.cs
文件 14929 2013-03-12 23:20 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\GameField.cs
文件 3830 2013-03-10 13:50 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\HuaDaBlock.csproj
文件 498 2013-03-10 13:37 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Program.cs
目录 0 2013-06-21 18:54 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Properties\
文件 1370 2013-03-10 09:39 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Properties\AssemblyInfo.cs
文件 2870 2013-03-10 09:39 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Properties\Resources.Designer.cs
文件 5612 2013-03-10 09:39 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Properties\Resources.resx
文件 1095 2013-03-10 09:39 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Properties\Settings.Designer.cs
文件 249 2013-03-10 09:39 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\Properties\Settings.settings
目录 0 2013-06-21 18:54 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\
目录 0 2013-06-21 21:07 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\
文件 3543552 2012-12-11 01:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\DevComponents.DotNetBar2.dll
文件 21504 2013-06-21 21:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\HuaDaBlock.exe
文件 50688 2013-06-21 21:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\HuaDaBlock.pdb
文件 11600 2013-06-21 21:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\HuaDaBlock.vshost.exe
文件 490 2010-03-17 22:39 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\HuaDaBlock.vshost.exe.manifest
文件 26112 2013-01-11 02:53 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\System.Data.ClientData.dll
文件 223744 2013-05-31 15:23 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\上万套源码免费下载.exe
文件 0 2013-05-02 01:55 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Debug\请勿用于商业用途 仅供测试 技术交流
目录 0 2013-06-21 21:07 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\bin\Release\
目录 0 2013-06-21 18:54 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\obj\
目录 0 2013-06-21 18:54 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\obj\x86\
目录 0 2013-06-21 21:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\obj\x86\Debug\
文件 5185 2013-06-21 21:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\obj\x86\Debug\DesignTimeResolveAssemblyReferences.cache
文件 6481 2013-06-21 21:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\obj\x86\Debug\DesignTimeResolveAssemblyReferencesInput.cache
文件 1026 2013-06-21 21:06 C#俄罗斯方块(使用VS2010编写)\HuaDaBlock\HuaDaBlock\obj\x86\Debug\GenerateResource.read.1.tlog
............此处省略13个文件信息
相关资源
- C# TIP文件生成和拆解
- C#解析HL7消息的库135797
- C# OCR数字识别实例,采用TessnetOcr,对
- 考试管理系统 - C#源码
- asp.net C#购物车源代码
- C#实时网络流量监听源码
- C#百度地图源码
- Visual C#.2010从入门到精通配套源程序
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
评论
共有 条评论