资源简介
上班闲着无聊做了一个JAVA版的GUI计时器,包括了中午的打卡时间、下午的下班时间、周末的倒计时和当天已加班的时间。后续又整合了国内各个能放假的节日倒计时(包括春节、中秋等农历节日)。最后 实在是闲着没事 做了贪吃蛇和扫雷两款小游戏整合到里面 闲着没事 打发打发时间 有兴趣的可以拿去参考参考
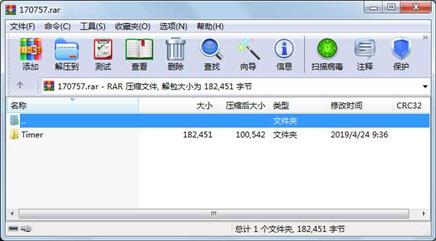
代码片段和文件信息
package com.yf.ui;
import java.awt.Color;
import java.awt.Container;
import java.awt.GridLayout;
import java.awt.Image;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.Jframe;
import javax.swing.JOptionPane;
public class Mine implements ActionListener {
Jframe frame = new Jframe(“扫雷“);
JButton reset = new JButton(“重新开始“);
Container container = new Container();
// 游戏数据结构
int rowG = 20;
int colG = 20;
int leiCountG = 50;
JButton[][] buttons = new JButton[rowG][colG];
int[][] counts = new int[rowG][colG];
final int LEICODE = 99;
// 构造函数
/**
* @wbp.parser.entryPoint
*/
public Mine() {
// 1、设置窗口
frame.setSize(900 800);
frame.setResizable(false);// 是否可改变窗口大小
frame.setDefaultCloseOperation(Jframe.DISPOSE_ON_CLOSE);
Image img = Toolkit.getDefaultToolkit().getImage(“config/lei.jpg“);// 窗口图标
frame.setIconImage(img);
// 2、添加重来按钮
addResetButton();
// 添加按钮
addButtons();
// 埋雷
addLei();
// 添加雷的计算
calcNeiboLei();
frame.setVisible(true);
}
public void addResetButton() { //重来按钮
reset.setBounds(385 7 122 23);
reset.setOpaque(true);
reset.addActionListener(this);
frame.getContentPane().setLayout(null);
frame.getContentPane().add(reset);
}
public void addLei() { //添加雷
Random rand = new Random();
int randRow randCol;
for (int i = 0; i < leiCountG; i++) {
randRow = rand.nextInt(rowG);
randCol = rand.nextInt(colG);
if (counts[randRow][randCol] == LEICODE) {
i--;
} else {
counts[randRow][randCol] = LEICODE;
// buttons[randRow][randCol].setText(“*“);
}
}
}
public void addButtons() { //初始化界面
container.setBounds(10 36 874 725);
frame.getContentPane().add(container);
container.setLayout(new GridLayout(rowG colG));
for (int i = 0; i < rowG; i++) {
for (int j = 0; j < colG; j++) {
JButton button = new JButton();
button.setBackground(Color.GRAY);
button.setOpaque(true);
button.addActionListener(this);
buttons[i][j] = button;
container.add(button);
}
}
}
public void calcNeiboLei() {
int count;
for (int i = 0; i < rowG; i++) {
for (int j = 0; j < colG; j++) {
count = 0;
if (counts[i][j] == LEICODE)
continue;
if (i > 0 && j > 0 && counts[i - 1][j - 1] == LEICODE)
count++;
if (i > 0 && counts[i - 1][j] == LEICODE)
count++;
if (i > 0 && j < 19 && counts[i - 1][j + 1] == LEICODE)
count++;
if (j > 0 && counts[i][j - 1] == LEICODE)
count++;
if (j < 19 && counts[i][j + 1] == LEICODE)
count++;
if (i < 19 && j > 0 && counts[i + 1][j - 1] == LEICODE)
count++;
if (i < 19 && counts[i + 1][j] == LEICODE)
count++;
if (i < 19 && j < 19 && counts[i + 1][j + 1] == LEICODE)
count++;
counts[i][j] = count;
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 2779 2019-04-22 15:30 Timer\.classpath
文件 381 2019-04-22 15:30 Timer\.project
文件 57 2019-04-22 16:00 Timer\.settings\org.eclipse.core.resources.prefs
文件 598 2019-04-22 15:30 Timer\.settings\org.eclipse.jdt.core.prefs
文件 5715 2019-04-28 13:47 Timer\bin\com\yf\ui\Mine.class
文件 2308 2019-04-28 13:47 Timer\bin\com\yf\ui\Snake$1.class
文件 2296 2019-04-28 13:47 Timer\bin\com\yf\ui\Snake$2.class
文件 1906 2019-04-28 13:47 Timer\bin\com\yf\ui\Snake$Timer.class
文件 9310 2019-04-28 13:47 Timer\bin\com\yf\ui\Snake.class
文件 366 2019-04-28 13:47 Timer\bin\com\yf\ui\Tile.class
文件 869 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$1.class
文件 733 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$2.class
文件 1197 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$3.class
文件 1197 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$4.class
文件 1197 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$5.class
文件 1197 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$6.class
文件 850 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$7$1.class
文件 1783 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$7.class
文件 1892 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$8.class
文件 1042 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication$9.class
文件 8315 2019-04-28 13:47 Timer\bin\com\yf\ui\TimerApplication.class
文件 2619 2019-04-28 13:47 Timer\bin\com\yf\util\AePlayWave.class
文件 363 2019-04-28 13:47 Timer\bin\com\yf\util\Lunar.class
文件 8617 2019-04-28 13:47 Timer\bin\com\yf\util\LunarSolarConverter.class
文件 342 2019-04-28 13:47 Timer\bin\com\yf\util\Solar.class
文件 8330 2019-04-30 16:28 Timer\bin\com\yf\util\TimerUtil.class
文件 12506 2019-04-23 14:30 Timer\config\eat.wav
文件 12858 2019-04-23 14:40 Timer\config\icon.jpg
文件 1561 2019-04-24 10:04 Timer\config\lei.jpg
文件 35402 2019-04-23 14:30 Timer\config\over.wav
............此处省略23个文件信息
相关资源
- 微博系统(Java源码,servlet+jsp),适
- java串口通信全套完整代码-导入eclip
- jsonarray所必需的6个jar包.rar
- 三角网构TIN生成算法,Java语言实现
- java代码编写将excel数据导入到mysql数据
- Java写的cmm词法分析器源代码及javacc学
- JAVA JSP公司财务管理系统 源代码 论文
- JSP+MYSQL旅行社管理信息系统
- 推荐算法的JAVA实现
- 基于Java的酒店管理系统源码(毕业设
- java-图片识别 图片比较
- android毕业设计
- java23种设计模式+23个实例demo
- java Socket发送/接受报文
- JAVA828436
- java界面美化 提供多套皮肤直接使用
- 在线聊天系统(java代码)
- 基于Java的图书管理系统807185
- java中实现将页面数据导入Excel中
- java 企业销售管理系统
- java做的聊天系统(包括正规课程设计
- Java编写的qq聊天室
- 商店商品管理系统 JAVA写的 有界面
- JAVA开发聊天室程序
- 在linux系统下用java执行系统命令实例
- java期末考试试题两套(答案) 选择(
- JAVA3D编程示例(建模、交互)
- Java 文件加密传输
- java做的房产管理系统
- 基于jsp的bbs论坛 非常详细
评论
共有 条评论