资源简介
RSA非对称加密,指定一个密码种子,使用该密码种子用java生成密钥对,并把公钥分发到客户端(浏览器),保存密码种子;JS采用公钥对重要信息进行加密,然后传回后台,取出密码种子重新生成密码对,使用私钥对密文进行解密。要保证密码对的安全就必须保证密码种子的不可预知性。
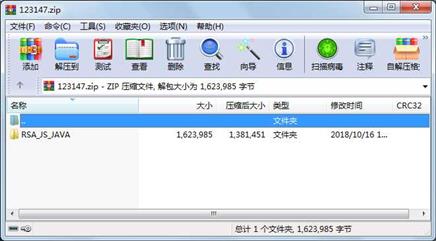
代码片段和文件信息
package org.common.util;
import java.security.InvalidParameterException;
import java.security.KeyFactory;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
import java.security.PrivateKey;
import java.security.Provider;
import java.security.SecureRandom;
import java.security.interfaces.RSAPrivateKey;
import java.security.interfaces.RSAPublicKey;
import javax.crypto.Cipher;
import org.apache.commons.lang3.StringUtils;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import org.bouncycastle.util.encoders.Hex;
/**
* RSA算法加密/解密工具类。
*
* @author Administrator
*
*/
public class RSA {
/** 算法名称 */
private static final String ALGORITHOM = “RSA“;
/** 密钥大小 */
private static final int KEY_SIZE = 1024;
/** 默认的安全服务提供者 */
private static final Provider DEFAULT_PROVIDER = new BouncyCastleProvider();
private static KeyPairGenerator keyPairGen = null;
private static KeyFactory keyFactory = null;
/** 缓存的密钥对。 */
private static KeyPair oneKeyPair = null;
private static String radamKey = ““;
static {
try {
keyPairGen = KeyPairGenerator.getInstance(ALGORITHOM
DEFAULT_PROVIDER);
keyFactory = KeyFactory.getInstance(ALGORITHOM DEFAULT_PROVIDER);
} catch (NoSuchAlgorithmException ex) {
ex.printStackTrace();
}
}
/**
* 生成并返回RSA密钥对。
*/
private static synchronized KeyPair generateKeyPair() {
try {
keyPairGen.initialize(KEY_SIZE
new SecureRandom(radamKey.getBytes()));
oneKeyPair = keyPairGen.generateKeyPair();
return oneKeyPair;
} catch (InvalidParameterException ex) {
ex.printStackTrace();
} catch (NullPointerException ex) {
ex.printStackTrace();
}
return null;
}
/** 返回已初始化的默认的公钥。 */
public static RSAPublicKey getDefaultPublicKey() {
KeyPair keyPair = generateKeyPair();
if (keyPair != null) {
return (RSAPublicKey) keyPair.getPublic();
}
return null;
}
/**
* 使用指定的私钥解密数据。
*
* @param privateKey 给定的私钥。
* @param data 要解密的数据。
* @return 原数据。
*/
public static byte[] decrypt(PrivateKey privateKey byte[] data) throws Exception {
Cipher ci = Cipher.getInstance(ALGORITHOM DEFAULT_PROVIDER);
ci.init(Cipher.DECRYPT_MODE privateKey);
return ci.doFinal(data);
}
/**
* 使用默认的私钥解密给定的字符串。
*
* 若{@code encrypttext} 为 {@code null}或空字符串则返回 {@code null}。
* 私钥不匹配时,返回 {@code null}。
*
* @param encrypttext 密文。
* @return 原文字符串。
*/
public static String decryptString(String encrypttext) {
if(StringUtils.isBlank(encrypttext)) {
return null;
}
KeyPair keyPair = generateKeyPair();
try {
byte[] en_data = Hex.decode(encrypttext);
byte[] data = decrypt((RSAPrivateKey)keyPair.getPrivate() en_data);
return new String(data);
} c
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2018-10-16 17:07 RSA_JS_JAVA\
目录 0 2013-06-13 22:08 RSA_JS_JAVA\RSA_JS_JAVA\
文件 1598015 2009-05-01 14:12 RSA_JS_JAVA\RSA_JS_JAVA\bouncycastle.jar
文件 932 2013-06-13 22:12 RSA_JS_JAVA\RSA_JS_JAVA\readme.txt
文件 1319 2013-06-10 13:10 RSA_JS_JAVA\RSA_JS_JAVA\rsa.html
文件 4641 2013-06-13 22:09 RSA_JS_JAVA\RSA_JS_JAVA\RSA.java
文件 19078 2012-01-11 11:59 RSA_JS_JAVA\RSA_JS_JAVA\security.js
相关资源
- Java 文件加密传输
- C#和Java实现互通的RSADES加解密算法
- RSA数字签名算法的具体实现
- Rsa非对称加密的Java实现和举例更新版
- 完美使用RSA2结合AES对数据进行加密兼
- Android RSA加密jar包
- RSA算法JAVA公钥加密,C#私钥解密
- 支付宝RSA加解密工具
- RSA加密登录示例278136
- Android RSA加密解密文件
- RSA加密传输AES的key和iv js加密 java解
- RSA加密解密 JS加密 JAVA解密
- C# RSA加密、支持JAVA格式公钥私钥
- 基于JAVA的RSA文件加密软件的设计与实
- javaweb使用rsa加密解密jar包
- RSA加解密源码及测试代码完整java工程
- JAVA的综合加解密聊天程序,附带文档
- MD5和RSA加密算法Java完成实现
- RSA前台加密后台解密Demo
- Java非对称加密源代码(RSA)-测试包
- android数据传输RSA加密DEMO
- javaRSA加密C++RSA解密
- android加密
- 安卓手机通用adb驱动UniversalAdbDriver官
- javafx_scenebuilder-2_0-macosx-universal.dmg
- RSA加密登录
- java实现简单RSA 公钥密码系统 源代码
- RSA算法与DES算法的实现
- Java 生成RSA密钥进行数据加密解密 支
- RSA加密解密java
评论
共有 条评论