资源简介
Android记账源代码
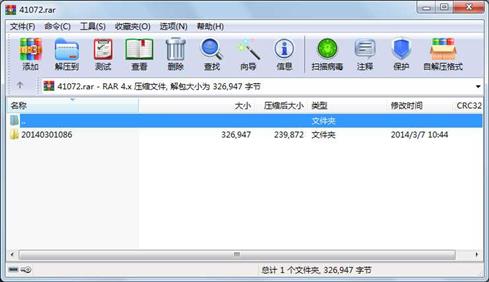
代码片段和文件信息
// Copyright 2013 Square Inc.
package com.squareup.timessquare;
import android.content.Context;
import android.util.AttributeSet;
import android.widget.TextView;
import com.squareup.timessquare.MonthCellDescriptor.RangeState;
public class CalendarCellView extends TextView {
private static final int[] STATE_SELECTABLE = {
R.attr.state_selectable
};
private static final int[] STATE_CURRENT_MONTH = {
R.attr.state_current_month
};
private static final int[] STATE_TODAY = {
R.attr.state_today
};
private static final int[] STATE_HIGHLIGHTED = {
R.attr.state_highlighted
};
private static final int[] STATE_RANGE_FIRST = {
R.attr.state_range_first
};
private static final int[] STATE_RANGE_MIDDLE = {
R.attr.state_range_middle
};
private static final int[] STATE_RANGE_LAST = {
R.attr.state_range_last
};
private boolean isSelectable = false;
private boolean isCurrentMonth = false;
private boolean isToday = false;
private boolean isHighlighted = false;
private RangeState rangeState = RangeState.NONE;
@SuppressWarnings(“UnusedDeclaration“)
public CalendarCellView(Context context AttributeSet attrs) {
super(context attrs);
}
public void setSelectable(boolean isSelectable) {
this.isSelectable = isSelectable;
refreshDrawableState();
}
public void setCurrentMonth(boolean isCurrentMonth) {
this.isCurrentMonth = isCurrentMonth;
refreshDrawableState();
}
public void setToday(boolean isToday) {
this.isToday = isToday;
refreshDrawableState();
}
public void setRangeState(MonthCellDescriptor.RangeState rangeState) {
this.rangeState = rangeState;
refreshDrawableState();
}
public void setHighlighted(boolean highlighted) {
isHighlighted = highlighted;
}
@Override protected int[] onCreateDrawableState(int extraSpace) {
final int[] drawableState = super.onCreateDrawableState(extraSpace + 5);
if (isSelectable) {
mergeDrawableStates(drawableState STATE_SELECTABLE);
}
if (isCurrentMonth) {
mergeDrawableStates(drawableState STATE_CURRENT_MONTH);
}
if (isToday) {
mergeDrawableStates(drawableState STATE_TODAY);
}
if (isHighlighted) {
mergeDrawableStates(drawableState STATE_HIGHLIGHTED);
}
if (rangeState == MonthCellDescriptor.RangeState.FIRST) {
mergeDrawableStates(drawableState STATE_RANGE_FIRST);
} else if (rangeState == MonthCellDescriptor.RangeState.MIDDLE) {
mergeDrawableStates(drawableState STATE_RANGE_MIDDLE);
} else if (rangeState == RangeState.LAST) {
mergeDrawableStates(drawableState STATE_RANGE_LAST);
}
return drawableState;
}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
....... 142 2014-01-28 22:42 20140301086\android-times-square-master\.gitignore
....... 388 2014-01-28 22:42 20140301086\android-times-square-master\.travis.yml
....... 1010 2014-01-28 22:42 20140301086\android-times-square-master\CHANGELOG.md
....... 5074 2014-01-28 22:42 20140301086\android-times-square-master\checkst
....... 737 2014-01-28 22:42 20140301086\android-times-square-master\CONTRIBUTING.md
....... 292 2014-01-28 22:42 20140301086\android-times-square-master\library\AndroidManifest.xm
....... 2591 2014-01-28 22:42 20140301086\android-times-square-master\library\pom.xm
....... 382 2014-01-28 22:42 20140301086\android-times-square-master\library\project.properties
....... 699 2014-01-28 22:42 20140301086\android-times-square-master\library\res\color\calendar_text_selector.xm
....... 951 2014-01-28 22:42 20140301086\android-times-square-master\library\res\drawable\calendar_bg_selector.xm
....... 2555 2014-01-28 22:42 20140301086\android-times-square-master\library\res\layout\month.xm
....... 1577 2014-01-28 22:42 20140301086\android-times-square-master\library\res\layout\week.xm
....... 510 2014-01-28 22:42 20140301086\android-times-square-master\library\res\values\attrs.xm
....... 689 2014-01-28 22:42 20140301086\android-times-square-master\library\res\values\colors.xm
....... 340 2014-01-28 22:42 20140301086\android-times-square-master\library\res\values\dimens.xm
....... 277 2014-01-28 22:42 20140301086\android-times-square-master\library\res\values\strings.xm
....... 1083 2014-01-28 22:42 20140301086\android-times-square-master\library\res\values\st
....... 156 2014-01-28 22:42 20140301086\android-times-square-master\library\res\values-v9\strings.xm
....... 2723 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\CalendarCellView.java
....... 4326 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\CalendarGridView.java
....... 28675 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\CalendarPickerView.java
....... 2761 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\CalendarRowView.java
....... 406 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\Logr.java
....... 2102 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\MonthCellDesc
....... 859 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\MonthDesc
....... 3112 2014-01-28 22:42 20140301086\android-times-square-master\library\src\com\squareup\timessquare\MonthView.java
....... 23653 2014-01-28 22:42 20140301086\android-times-square-master\library\test\com\squareup\timessquare\CalendarPickerViewTest.java
....... 11358 2014-01-28 22:42 20140301086\android-times-square-master\LICENSE.txt
....... 4686 2014-01-28 22:42 20140301086\android-times-square-master\pom.xm
....... 2566 2014-01-28 22:42 20140301086\android-times-square-master\README.md
............此处省略55个文件信息
相关资源
- android毕业设计
- 百度地图自定义Markerandroid
- Android分区工具包
- android-support-v4.jar已打包进去源代码
- u-blox_Android_GNSS_Driver_v3.10驱动源码+中
- 个人根据Android移动开发案例详解手写
- android 视频播放器 项目和原码
- Android【动画】【特效】 17种动画特效
- 基于Android智能家居详细设计(经典)
- android通过JDBC连接Mysql数据库
- Android通讯录的源代码
- android 瀑布流Demo
- 指纹传感器FPC1080在android下的驱动
- delphi xe5 android 调用照相机摄像头拍照
- Android手机连连看游戏源码
- android-sdk-windows v2.3离线完整版
- android 底部弹出菜单(带透明背景)
- Android工程模式简介.rar
- Android蓝牙和Cors网络开发源码
- Android powermanger wakelock
- Android v7的一些jar包
- 最新android supportV7包
- android图片压缩工具类分享
- 单机搭建Android(解决Network is unreach
- Android上监听收到的短信(SMS)
- android电商app源码
- Android代码-多功能拨号盘源码.zip
- printershare直接破解版--11.5(适配andr
- android RDP远程桌面客户端源码
- 手机远程控制手机android
评论
共有 条评论