资源简介
sift图像匹配
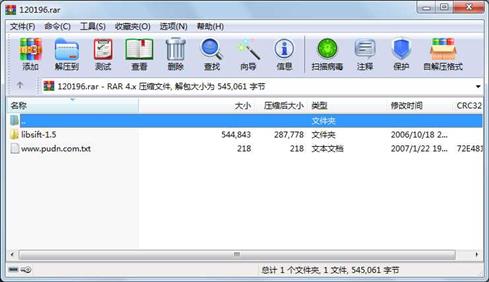
代码片段和文件信息
/* AreaFilter.cs
*
* Area maximizing match filtration using convex hull and polygon-area
* maximization.
*
* (C) Copyright 2004 -- Sebastian Nowozin (nowozin@cs.tu-berlin.de)
*
* Convex hull code based on Ken Clarksons original C implementation.
* Polygon area formula by Paul Bourke.
*/
using System;
using System.Collections;
public class
AreaFilter
{
public static void Main (string[] args)
{
ArrayList al = new ArrayList ();
Random rnd = new Random ();
for (int n = 0 ; n < 10 ; ++n) {
Point p = new Point ();
p.x = rnd.NextDouble () * 400.0;
p.y = rnd.NextDouble () * 400.0;
al.Add (p);
}
foreach (Point p in al)
Console.WriteLine (“{0} {1} # GNUPLOT1“ p.x p.y);
AreaFilter conv = new AreaFilter ();
ArrayList hull = conv.CreateConvexHull (al);
Console.WriteLine (“\nhull: {0} elements“ hull.Count);
foreach (Point p in hull)
Console.WriteLine (“{0} {1} # GNUPLOT2“ p.x p.y);
Console.WriteLine (“\npolygon area: {0}“ conv.PolygonArea (hull));
}
public class Point
{
public double x y;
public object user = null; // Store anything for the user
}
// Measure the absolute area of a non self-intersecting polygon.
// Formula by Paul Bourke
// (http://astronomy.swin.edu.au/~pbourke/geometry/polyarea/)
//
// The input points must be ordered (clock- or counter-clockwise). The
// polygon is closed automatically between the first and the last point
// so there should not be two same points in the polygon point set.
public double PolygonArea (ArrayList orderedPoints)
{
double A = 0.0;
for (int n = 0 ; n < orderedPoints.Count ; ++n) {
Point p0 = (Point) orderedPoints[n];
Point p1 = (Point) orderedPoints[(n + 1) % orderedPoints.Count];
A += p0.x * p1.y - p1.x * p0.y;
}
A *= 0.5;
return (Math.Abs (A));
}
// Create the convex hull of a point set.
public ArrayList CreateConvexHull (ArrayList points)
{
return (CreateConvexHull ((Point[]) points.ToArray (typeof (Point))));
}
public ArrayList CreateConvexHull (Point[] points)
{
int chn = CreateHull (points);
ArrayList hull = new ArrayList ();
for (int k = 0 ; k < chn ; ++k)
hull.Add (points[k]);
return (hull);
}
public class CompareLow : IComparer {
public int Compare (object o1 object o2)
{
Point p1 = (Point) o1;
Point p2 = (Point) o2;
double v = p1.x - p2.x;
if (v > 0)
return (1);
if (v < 0)
return (-1);
v = p2.y - p1.y;
if (v > 0)
return (1);
if (v < 0)
return (-1);
// Equal point
return (0);
}
}
public class CompareHigh : IComparer {
CompareLow cl;
public CompareHigh ()
{
cl = new CompareLow ();
}
public int Compare (object o1 object o2)
{
return (cl.Compare (o2 o1));
}
}
private bool ccw (Point[] points int i int j int k)
{
double a = points[i].x - points[j].x;
double b = points[i].y - points[j].y;
double c = points[k].x - points[j].x;
double d = points[k].y - points[j].y;
return ((a * d
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 118784 2004-09-23 23:00 libsift-1.5\libsift-1.5\bin\ICSharpCode.SharpZipLib.dll
文件 129024 2004-09-23 23:00 libsift-1.5\libsift-1.5\bin\libsift.dll
文件 2337 2004-09-23 23:00 libsift-1.5\libsift-1.5\LICENSE
文件 2380 2004-09-23 23:00 libsift-1.5\libsift-1.5\README
文件 4225 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\AreaFilter.cs
文件 11074 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\BondBall.cs
文件 1448 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\distmetrictest.cs
文件 3899 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\GaussianConvolution.cs
文件 4236 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\ImageMap.cs
文件 7058 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\ImageMatchModel.cs
文件 17471 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\KDTree.cs
文件 4769 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\Keypointxm
文件 6959 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\LoweDetector.cs
文件 3053 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\Makefile
文件 26267 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\MatchKeys.cs
文件 4484 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\RANSAC.cs
文件 42026 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\ScaleSpace.cs
文件 4917 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\SimpleMatrix.cs
文件 765 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\TODO
文件 4563 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\Transform.cs
文件 4 2004-09-23 23:00 libsift-1.5\libsift-1.5\src\VERSION
文件 145100 2006-10-18 22:03 libsift-1.5\libsift-1.5.rar
目录 0 2006-10-18 22:03 libsift-1.5\libsift-1.5\bin
目录 0 2006-10-18 22:03 libsift-1.5\libsift-1.5\src
目录 0 2006-10-18 22:03 libsift-1.5\libsift-1.5
目录 0 2006-10-18 22:03 libsift-1.5
----------- --------- ---------- ----- ----
545061 27
- 上一篇:屏蔽迅雷7自动升级
- 下一篇:解决下拉菜单被if
rame遮住问题
评论
共有 条评论