资源简介
粒子系统表示三维计算机图形学中模拟一些特定的模糊现象的技术,而这些现象用其它传统的渲染技术难以实现的真实感的 game physics。经常使用粒子系统模拟的现象有火、爆炸、烟、水流、火花、落叶、云、雾、雪、尘、流星尾迹或者象发光轨迹这样的抽象视觉效果等等。
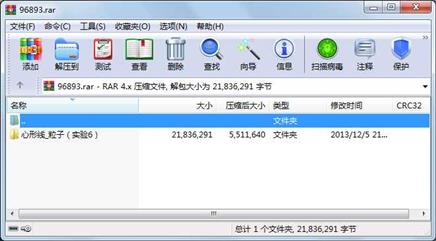
代码片段和文件信息
//=============================================================================
// Camera.cpp by Frank Luna (C) 2004 All Rights Reserved.
//=============================================================================
#include “Camera.h“
#include “DirectInput.h“
#include “d3dUtil.h“
#include “Terrain.h“
Camera* gCamera = 0;
Camera::Camera()
{
D3DXMatrixIdentity(&mView);
D3DXMatrixIdentity(&mProj);
D3DXMatrixIdentity(&mViewProj);
mPosW = D3DXVECTOR3(0.0f 0.0f 0.0f);
mRightW = D3DXVECTOR3(1.0f 0.0f 0.0f);
mUpW = D3DXVECTOR3(0.0f 1.0f 0.0f);
mLookW = D3DXVECTOR3(0.0f 0.0f 1.0f);
// Client should adjust to a value that makes sense for application‘s
// unit scale and the object the camera is attached to--e.g. car jet
// human walking etc.
mSpeed = 50.0f;
}
const D3DXMATRIX& Camera::view() const
{
return mView;
}
const D3DXMATRIX& Camera::proj() const
{
return mProj;
}
const D3DXMATRIX& Camera::viewProj() const
{
return mViewProj;
}
const D3DXVECTOR3& Camera::right() const
{
return mRightW;
}
const D3DXVECTOR3& Camera::up() const
{
return mUpW;
}
const D3DXVECTOR3& Camera::look() const
{
return mLookW;
}
D3DXVECTOR3& Camera::pos()
{
return mPosW;
}
void Camera::lookAt(D3DXVECTOR3& pos D3DXVECTOR3& target D3DXVECTOR3& up)
{
D3DXVECTOR3 L = target - pos;
D3DXVec3Normalize(&L &L);
D3DXVECTOR3 R;
D3DXVec3Cross(&R &up &L);
D3DXVec3Normalize(&R &R);
D3DXVECTOR3 U;
D3DXVec3Cross(&U &L &R);
D3DXVec3Normalize(&U &U);
mPosW = pos;
mRightW = R;
mUpW = U;
mLookW = L;
buildView();
buildWorldFrustumPlanes();
mViewProj = mView * mProj;
}
void Camera::setLens(float fov float aspect float nearZ float farZ)
{
D3DXMatrixPerspectiveFovLH(&mProj fov aspect nearZ farZ);
buildWorldFrustumPlanes();
mViewProj = mView * mProj;
}
void Camera::setSpeed(float s)
{
mSpeed = s;
}
bool Camera::isVisible(const AABB& box)const
{
// Test assumes frustum planes face inward.
D3DXVECTOR3 P;
D3DXVECTOR3 Q;
// N *Q *P
// | / /
// |/ /
// -----/----- Plane -----/----- Plane
// / / |
// / / |
// *P *Q N
//
// PQ forms diagonal most closely aligned with plane normal.
// For each frustum plane find the box diagonal (there are four main
// diagonals that intersect the box center point) that points in the
// same direction as the normal along each axis (i.e. the diagonal
// that is most aligned with the plane normal). Then test if the box
// is in front of the plane or not.
for(int i = 0; i < 6; ++i)
{
// For each coordinate axis x y z...
for(int j = 0; j < 3; ++j)
{
// Make PQ point in the same direction as the plane normal on this axis.
if( mFrustumPlanes[i][j] >= 0.0f )
{
P
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 591872 2013-12-05 21:18 心形线_粒子(实验6)\Debug\螺旋状粒子(实验6).exe
文件 2256728 2013-12-05 21:18 心形线_粒子(实验6)\Debug\螺旋状粒子(实验6).ilk
文件 3394560 2013-12-05 21:18 心形线_粒子(实验6)\Debug\螺旋状粒子(实验6).pdb
文件 699192 2005-09-25 11:23 心形线_粒子(实验6)\螺旋状粒子(实验6)\blend_hm1.dds
文件 7336 2005-11-30 23:01 心形线_粒子(实验6)\螺旋状粒子(实验6)\Camera.cpp
文件 1549 2005-11-30 22:58 心形线_粒子(实验6)\螺旋状粒子(实验6)\Camera.h
文件 11264 2005-12-23 13:11 心形线_粒子(实验6)\螺旋状粒子(实验6)\d3dApp.cpp
文件 2309 2005-07-29 12:41 心形线_粒子(实验6)\螺旋状粒子(实验6)\d3dApp.h
文件 5805 2005-10-11 10:31 心形线_粒子(实验6)\螺旋状粒子(实验6)\d3dUtil.cpp
文件 4585 2013-11-20 22:08 心形线_粒子(实验6)\螺旋状粒子(实验6)\d3dUtil.h
文件 8092 2013-12-05 21:18 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\BuildLog.htm
文件 94802 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\Camera.obj
文件 87753 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\d3dApp.obj
文件 424133 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\d3dUtil.obj
文件 68611 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\DirectInput.obj
文件 112142 2013-12-05 21:18 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\FireRingDemo.obj
文件 67381 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\GfxStats.obj
文件 239896 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\Heightmap.obj
文件 67 2013-12-05 21:18 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\mt.dep
文件 389661 2013-12-05 17:41 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\PSystem.obj
文件 468961 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\Terrain.obj
文件 945152 2013-12-05 21:18 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\vc90.idb
文件 659456 2013-12-05 21:18 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\vc90.pdb
文件 61799 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\Vertex.obj
文件 663 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\螺旋状粒子(实验6).exe.em
文件 728 2013-12-05 17:17 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\螺旋状粒子(实验6).exe.em
文件 621 2013-12-05 21:18 心形线_粒子(实验6)\螺旋状粒子(实验6)\Debug\螺旋状粒子(实验6).exe.intermediate.manifest
文件 2267 2005-06-30 19:29 心形线_粒子(实验6)\螺旋状粒子(实验6)\DirectInput.cpp
文件 1143 2005-06-30 22:02 心形线_粒子(实验6)\螺旋状粒子(实验6)\DirectInput.h
文件 131200 2005-09-10 11:18 心形线_粒子(实验6)\螺旋状粒子(实验6)\dirt.dds
............此处省略33个文件信息
- 上一篇:四个情感词典汇总.zip
- 下一篇:激光机原理以及应用简介.doc
相关资源
- Microsoft DirectX 8.0
- 20101028 DirectX(粒子系统改)
- DirectX 材质 模型 工具汉化版
- Directx 3D游戏 遥控飞机
- Dark GDK
- 运用DirectX9绘制太阳系
- Introduction to 3D Game Programming with Direc
- Directx12 游戏开发
- directx 10 龙书 中文版
- DirectX3D太阳系
- OPENGL粒子系统之喷泉
- DirectX.9.0SDK中文版帮助文档
- 屏幕融合软件源码
- Microsoft DirectX SDK (June 2010)
- DelphiX 2000.07.17 For D7 (DirectX)
- DirectX 太阳系
- directX8.0 SDK
- DirectX.Capture ffdshow摄像头录制视频拍照
- 《DirectX特效游戏程序设计》中文版全
- Jiggly Bubble 1.31.unitypackage
- 3Dmax 烟花
- Directx9 SDK
- 火焰渲染的粒子系统程序,单文档工
- [未整理]Direct X 11 3D 游戏开发编程基础
- DirectX版半条命MDL文件查看器
- DirectX 9.0 中文版传说中的龙书
- 实现三维图形绘制
- DirectX 龙书源码
- 太阳地球月亮旋转公转自转
- 基于Directx的VC视频捕获源代码.rar
评论
共有 条评论