资源简介
基于python2.7的LeNet5源代码实现,效果很不错。
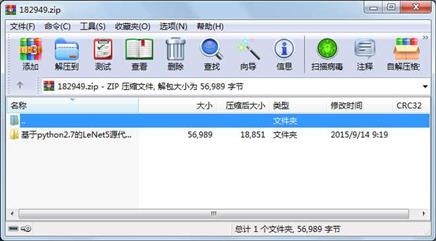
代码片段和文件信息
“““This tutorial introduces the LeNet5 neural network architecture
using Theano. LeNet5 is a convolutional neural network good for
classifying images. This tutorial shows how to build the architecture
and comes with all the hyper-parameters you need to reproduce the
paper‘s MNIST results.
This implementation simplifies the model in the following ways:
- LeNetConvPool doesn‘t implement location-specific gain and bias parameters
- LeNetConvPool doesn‘t implement pooling by average it implements pooling
by max.
- Digit classification is implemented with a logistic regression rather than
an RBF network
- LeNet5 was not fully-connected convolutions at second layer
References:
- Y. LeCun L. Bottou Y. Bengio and P. Haffner:
Gradient-based Learning Applied to Document
Recognition Proceedings of the IEEE 86(11):2278-2324 November 1998.
http://yann.lecun.com/exdb/publis/pdf/lecun-98.pdf
“““
import os
import sys
import time
import numpy
import theano
import theano.tensor as T
from theano.tensor.signal import downsample
from theano.tensor.nnet import conv
from logistic_sgd import LogisticRegression load_data
from mlp import Hiddenlayer
class LeNetConvPoollayer(object):
“““Pool layer of a convolutional network “““
def __init__(self rng input filter_shape image_shape poolsize=(2 2)):
“““
Allocate a LeNetConvPoollayer with shared variable internal parameters.
:type rng: numpy.random.RandomState
:param rng: a random number generator used to initialize weights
:type input: theano.tensor.dtensor4
:param input: symbolic image tensor of shape image_shape
:type filter_shape: tuple or list of length 4
:param filter_shape: (number of filters num input feature maps
filter height filter width)
:type image_shape: tuple or list of length 4
:param image_shape: (batch size num input feature maps
image height image width)
:type poolsize: tuple or list of length 2
:param poolsize: the downsampling (pooling) factor (#rows #cols)
“““
assert image_shape[1] == filter_shape[1]
self.input = input
# there are “num input feature maps * filter height * filter width“
# inputs to each hidden unit
fan_in = numpy.prod(filter_shape[1:])
# each unit in the lower layer receives a gradient from:
# “num output feature maps * filter height * filter width“ /
# pooling size
fan_out = (filter_shape[0] * numpy.prod(filter_shape[2:]) /
numpy.prod(poolsize))
# initialize weights with random weights
W_bound = numpy.sqrt(6. / (fan_in + fan_out))
self.W = theano.shared(
numpy.asarray(
rng.uniform(low=-W_bound high=W_bound size=filter_shape)
dtype=theano.config.floatX
)
borrow=True
)
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2015-09-14 09:19 基于python2.7的LeNet5源代码实现\
文件 12645 2015-08-20 22:08 基于python2.7的LeNet5源代码实现\convolutional_mlp.py
文件 20706 2015-08-20 22:08 基于python2.7的LeNet5源代码实现\convolutional_mlp_commentate.py
目录 0 2015-09-14 09:19 基于python2.7的LeNet5源代码实现\data\
文件 9457 2015-09-02 21:29 基于python2.7的LeNet5源代码实现\logistic_sgd.py
文件 14181 2015-08-20 22:08 基于python2.7的LeNet5源代码实现\mlp.py
相关资源
- 二级考试python试题12套(包括选择题和
- pywin32_python3.6_64位
- python+ selenium教程
- PycURL(Windows7/Win32)Python2.7安装包 P
- 英文原版-Scientific Computing with Python
- 7.图像风格迁移 基于深度学习 pyt
- 基于Python的学生管理系统
- A Byte of Python(简明Python教程)(第
- Python实例174946
- Python 人脸识别
- Python 人事管理系统
- 基于python-flask的个人博客系统
- 计算机视觉应用开发流程
- python 调用sftp断点续传文件
- python socket游戏
- 基于Python爬虫爬取天气预报信息
- python函数编程和讲解
- Python开发的个人博客
- 基于python的三层神经网络模型搭建
- python实现自动操作windows应用
- python人脸识别(opencv)
- python 绘图(方形、线条、圆形)
- python疫情卡UN管控
- python 连连看小游戏源码
- 基于PyQt5的视频播放器设计
- 一个简单的python爬虫
- csv文件行列转换python实现代码
- Python操作Mysql教程手册
- Python Machine Learning Case Studies
- python获取硬件信息
评论
共有 条评论