资源简介
本程序经历了华南赛区决赛的检验,通过此程序助我们顺利冲进国赛。
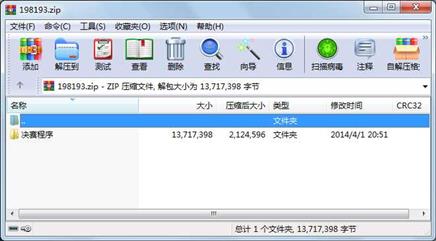
代码片段和文件信息
/*
* File: alloc.c
* Purpose: generic malloc() and free() engine
*
* Notes: 99% of this code stolen/borrowed from the K&R C
* examples.
*
*/
#include “common.h“
#include “stdlib.h“
#pragma section = “HEAP“
/********************************************************************/
/*
* This struct forms the minimum block size which is allocated and
* also forms the linked list for the memory space used with alloc()
* and free(). It is padded so that on a 32-bit machine all malloc‘ed
* pointers are 16-byte aligned.
*/
typedef struct ALLOC_HDR
{
struct
{
struct ALLOC_HDR *ptr;
unsigned int size;
} s;
unsigned int align;
unsigned int pad;
} ALLOC_HDR;
static ALLOC_HDR base;
static ALLOC_HDR *freep = NULL;
/********************************************************************/
void
free (void *ap)
{
ALLOC_HDR *bp *p;
bp = (ALLOC_HDR *)ap - 1; /* point to block header */
for (p = freep; !((bp > p) && (bp < p->s.ptr)) ; p = p->s.ptr)
{
if ((p >= p->s.ptr) && ((bp > p) || (bp < p->s.ptr)))
{
break; /* freed block at start or end of arena */
}
}
if ((bp + bp->s.size) == p->s.ptr)
{
bp->s.size += p->s.ptr->s.size;
bp->s.ptr = p->s.ptr->s.ptr;
}
else
{
bp->s.ptr = p->s.ptr;
}
if ((p + p->s.size) == bp)
{
p->s.size += bp->s.size;
p->s.ptr = bp->s.ptr;
}
else
{
p->s.ptr = bp;
}
freep = p;
}
/********************************************************************/
void *
malloc (unsigned nbytes)
{
/* Get addresses for the HEAP start and end */
#if (defined(CW))
extern char __HEAP_START;
extern char __HEAP_END[];
#elif (defined(IAR))
char *__HEAP_START = __section_begin(“HEAP“);
char *__HEAP_END = __section_end(“HEAP“);
#endif
ALLOC_HDR *p *prevp;
unsigned nunits;
nunits = ((nbytes + sizeof(ALLOC_HDR) - 1) / sizeof(ALLOC_HDR)) + 1;
if ((prevp = freep) == NULL)
{
p = (ALLOC_HDR *)__HEAP_START;
p->s.size = ( ((uint32)__HEAP_END - (uint32)__HEAP_START)
/ sizeof(ALLOC_HDR) );
p->s.ptr = &base;
base.s.ptr = p;
base.s.size = 0;
prevp = freep = &base;
}
for (p = prevp->s.ptr; ; prevp = p p = p->s.ptr)
{
if (p->s.size >= nunits)
{
if (p->s.size == nunits)
{
prevp->s.ptr = p->s.ptr;
}
else
{
p->s.size -= nunits;
p += p->s.size;
p->s.size = nunits;
}
freep = prevp;
return (void *)(p + 1);
}
if (p == freep)
return NULL;
}
}
/**************************************************
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2014-04-01 20:51 决赛程序\
文件 171 2014-02-22 11:45 决赛程序\TEST1.eww
目录 0 2014-04-01 20:51 决赛程序\build\
目录 0 2014-11-05 20:15 决赛程序\build\TEST1\
目录 0 2014-04-01 20:51 决赛程序\build\TEST1\Debug\
目录 0 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Exe\
文件 60551 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Exe\fire_gpio_demo.hex
文件 305708 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Exe\fire_gpio_demo.out
文件 21559 2014-07-28 15:38 决赛程序\build\TEST1\Debug\Exe\fire_gpio_demo.sim
目录 0 2014-07-28 15:34 决赛程序\build\TEST1\Debug\List\
文件 36111 2014-07-28 15:34 决赛程序\build\TEST1\Debug\List\fire_gpio_demo.map
目录 0 2014-11-05 20:15 决赛程序\build\TEST1\Debug\Obj\
文件 21864 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\FTM.o
文件 43508 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\LCDinit.o
文件 14348 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\LED.o
文件 9812 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\PIT.o
文件 2361 2014-08-17 13:34 决赛程序\build\TEST1\Debug\Obj\TEST1.pbd
文件 26584 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\adc.o
文件 11840 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\alloc.o
文件 17044 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\arm_cm4.o
文件 8656 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\assert.o
文件 54868 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\chu_li.o
文件 1760 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\crt0.o
文件 27876 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\data.o
文件 9312 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\delay.o
文件 22188 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\dma.o
文件 8552 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\exti.o
文件 18180 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\gpio.o
文件 10440 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\io.o
文件 19212 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\isr.o
文件 9704 2014-07-28 15:34 决赛程序\build\TEST1\Debug\Obj\key.o
............此处省略249个文件信息
相关资源
- 飞思卡尔单片机MC9S12XS12G128驱动(硬件
- 飞思卡尔68HC08Metrowerks_CodeWarrior开发软
- 飞思卡尔单片机.s19文件方法详解
- 科来杯山东省大学生网络安全技能大
- 滤波-卡尔曼滤波-互补滤波
- 实现飞思卡尔两轮智能车的直立芯片
- 飞思卡尔智能车摄像头组
- 飞思卡尔编程入门菜鸟教程
- 飞思卡尔所有的算法
- 2017年深圳杯建模挑战赛C题决赛论文独
- 飞思卡尔电磁程序
- 飞思卡尔kl25参考程序
- 飞思卡尔小车仿真软件
- CCP源码、驱动和协议详解 包含了飞思
- 飞思卡尔 摄像头 可跑程序 完整的国
- 第十三届电磁组程序 -.rar
- 飞思卡尔软件教程资料
- 飞思卡尔智能车入门资料大全.7z
- 飞思卡尔HCS08中文手册
- 飞思卡尔KEA128核心系统原理图和封装
- 飞思卡尔MC9S08DZ60单片机学习板说明书
- 飞思卡尔P2020平台介绍
- 飞思卡尔各种芯片功详解
- 飞思卡尔BTN7971双电机驱动模块
- 飞思卡尔MPC5125参考手册
- 飞思卡尔S12系列单片机系统硬件设计
- 飞思卡尔MC9S12单片机完整版说明书(
- 破解版Visualscope及其使用方法,直立车
- MC9S12DG128各模块例程飞思卡尔
- 飞思卡尔MC9S12XS128---编程指导---自己积
评论
共有 条评论